20 Jan 2024
This whole time I thought I needed to do this for supporting files in forms:
form_with(model: attachment, html: { enctype: "multipart/form-data"} )
but it turns out you can actually just do this:
form_with(model: attachment, multipart: true)
which is cleaner and easier to remember.
03 Jan 2024
Indexes are something I don’t really think about a lot, but know that they can have dramatic impacts on performance.
A good rule of thumb is to create database indexes for everything that is referenced in the WHERE, HAVING and ORDER BY parts of your SQL queries.
— Igor Šarčević
You should pretty much add indexes to all foreign keys.
An index for a certain column/columns in a database works similarly to an index in a book. Instead of scanning every page of a book for all instances of a subject, we flip to the index, which is usually alphabetized in some fashion, and find the subject in there. The subject entry points us to the relevant pages of the book.
— source
Another case is for uniqueness.
For example, if a users table has a uniqueness validation for combination email and username.
(i.e. there cannot be more than one user with the same email/username combo)
Indexing the email attribute will allow our database to abort any save operation on a non-unique email, giving us a second line of defense against the wild users of your site.
add_index :table_name, [:column_name_a, :column_name_b], unique: true
source
30 Nov 2023
Whenever I’ve had a predifined class and wanted to add/modify a method to it, I’ve always patched it or seen people make a Concern or something.
Apparently, Ruby has a built in thing for this exactly that is better called Refinements.
# Instead of a monkey patch
class String
def to_a
return [] if blank?
str = dup
str.slice!("[")
str.slice!("]")
str.split(",")
end
end
# Do this instead
module StringToArray
refine String do
def to_a
return [] if blank?
str = dup
str.slice!("[")
str.slice!("]")
str.split(",")
end
end
end
# Then in module or class that you use it
module ResourceCreator
using StringToArray
end
30 Nov 2023
Binary search through your git log to find where you hecked up and introduced a bug.
This command saved my life. I was trying to debug a really silly error that I couldn’t debug with error messages because the issue happened because a method was added to a core class and the stacktrace was not helpful. git bisect
was the ONLY thing that worked.
Video I watched:
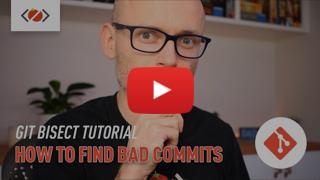
Notes
git bisect start
git bisect bad # marks current commit doesn't work
git bisect good d0bf3f6 # specify latest commit that *does* work
# for each commit given, mark bad or good with
git bisect bad
git bisect good
# when done,
git bisect reset
Aside: embed videos in markdown with: https://markdown-videos.jorgenkh.no/
16 Oct 2023
For context, I was working on a feature to to allow users to preview the associated thread (in a modal) of each notification from the notifications index page. While the notifications were paginated, the post threads could be very large. The naive approach of just having a modal for each notification was very ineffecient when threads were large and made the page load slow.
To increase the performace, I wanted to render just one modal and have the content change depending on which notification was clicked.
This was a little tricky to get timings right for when and what content to load, but this article helped me a lot.
https://philonrails.substack.com/p/loading-dynamic-content-on-opening
Eventually I added an auto-scroll to the exact part of the thread that was related to the notification to.