21 Aug 2023
TIL you can find the intersection of two Arrays easily with &
.
a = [18, 22, 33, 4, 5, 6]
b = [5, 4, 22, 1, 88, 9]
c = [18, 22, 33, 40, 50, 6]
# a intersecting b
puts "intersection of a and b : #{a & b}\n\n"
# => intersection of a and b : [22, 4, 5]
# a intersecting c
puts "intersection of a and c : #{a & c}\n\n"
# =>intersection of a and c : [18, 22, 33, 6]
# b intersecting c
puts "intersection of b and c : #{b & c}\n\n"
# => intersection of b and c : [22]
— Source
02 Aug 2023
Ran into a hard-to-debug error where a nav link was unable to be clicked on because it wasn’t “visible”. Printing the HTML of the page and turning off headless proved to me that the link was both present and visible, so I was confused for a while.
Apparently, the default browser size for headless chrome is Something like 800, 600
which almost certainly will activate a responsive layout that could cause some elements (like navbar links) to become “invisible”.
The browser has a wider default size in non-headless mode, so it was not immediately obvious to me that an element could be invisible when the browser is headless.
This makes me think that it should be best practice to always specify a default browser size for tests 🤔
06 Jul 2023
I learned about a few useful methods that I hadn’t heard of before.
reflect_on_association
This method allows you to check if an association exists, without making a database query.
- Will return
nil
if no associated records are found.
- Will return an
AggregateReflection
object if records are found.
class Post < ActiveRecord::Base
has_many :upvotes
end
class Upvote < ActiveRecord::Base
belongs_to :post
end
Post.reflect_on_association(:upvotes)
Useful if you need to get all associated objects from an ActiveRecord::Relation
.
Assuming:
class Author < ActiveRecord::Base
has_many :books
end
class Book < ActiveRecord::Base
belongs_to :author
end
and we want to get all the books from a list of authors…
Normally, my first thought we be to do something like this:
Author.all.includes(:books).map(&:books)
but there is a more readable way to do this with extract_associated
.
Author.all.extract_associated(:books)
which actually does something similar, so the benefit is mostly the readability.
missing
Ever need to find records with no associated records?
For example:
class City < ActiveRecord::Base
has_many :photos
end
class Photo < ActiveRecord::Base
belongs_to :city
end
if I want to find all cities that have no photos, previously, I thought you needed to do something like this:
City.includes(:photos).where(photos: { city_id: nil })
missing
does this.
City.where.missing(:photos)
associated
New in Rails 7. Sort of the inverse of missing
, but associated
checks for the presence of an association.
Finding all the cities that have photos is as simple as:
City.where.associated(:photos)
03 Jul 2023
I know people say never to use floats anymore, but surely they can still be used for whatever they were originally intended for… right?
The float CSS property places an element on the left or right side of its container, allowing text and inline elements to wrap around it. The element is removed from the normal flow of the page, though still remaining a part of the flow (in contrast to absolute positioning).
— MDN Docs
Seems unique enough. I don’t think flexbox or CSS grid (the modern layout techniques) do a better job at this.
The float property was introduced to allow web developers to implement layouts involving an image floating inside a column of text, with the text wrapping around the left or right of it. The kind of thing you might get in a newspaper layout.
So the original purpose was to position images within text in a few specific ways.
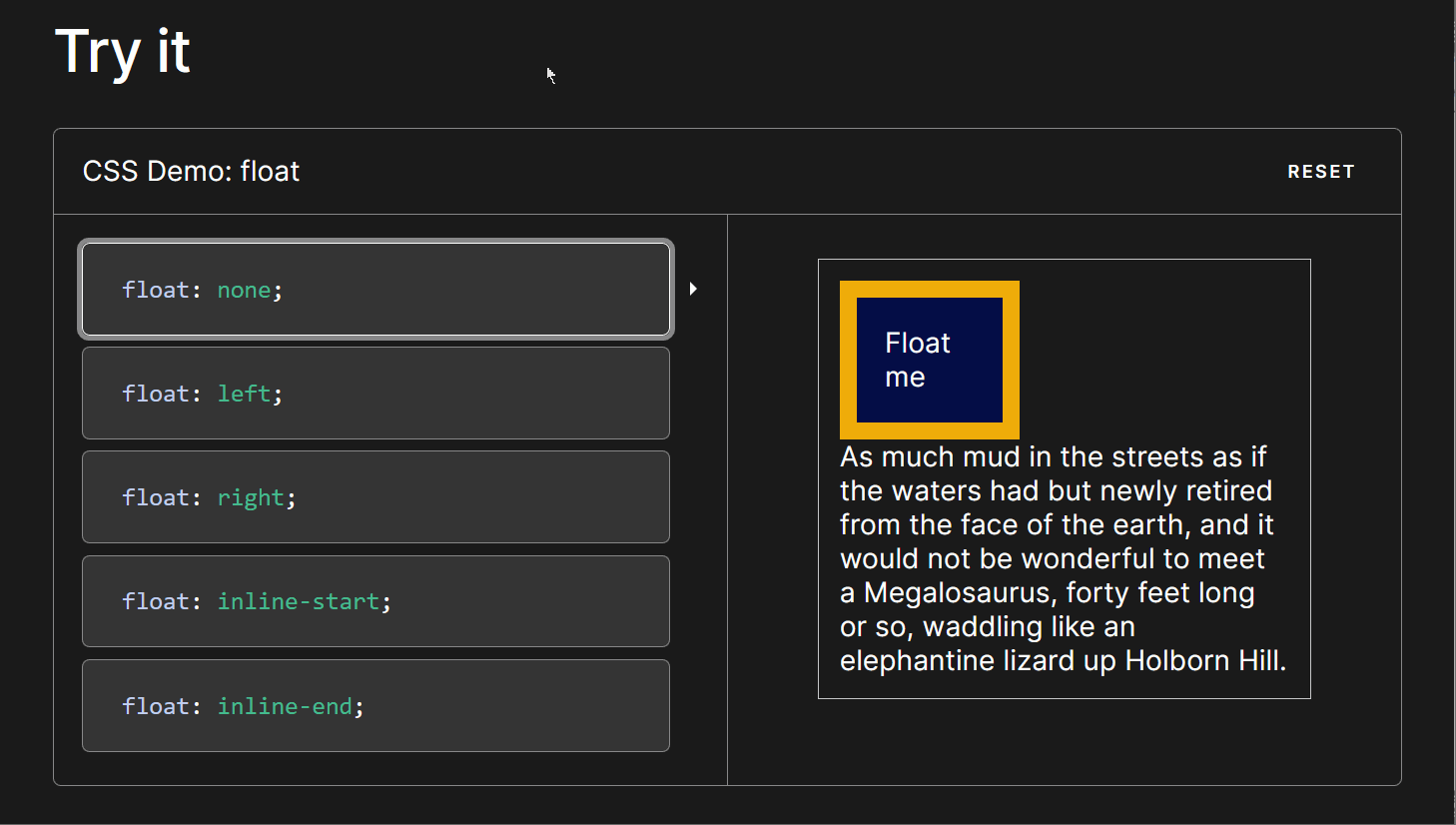
<div>
<div>Float me</div>
As much mud in the streets as if the waters had but newly retired from the face of the earth, and it would not be wonderful to meet a Megalosaurus, forty feet long or so, waddling like an elephantine lizard up Holborn Hill.
</div>
More depth on floats here.
30 Jun 2023
When using a URL helper you can specify a fragment with the anchor
option:
post_path(post.root, anchor: "post_#{post.id}")
# => "/posts/42#post_69
Previously, I was manually doing something similar like:
"#{post_path(post.root)}#post_#{post.id}"
# => "/posts/42#post_69